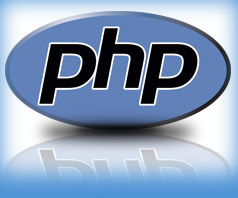
Variables in PHP are identical to variables in most other programming languages. For the uninitiated, a variable can be thought of as a name that’s given to an imaginary box into which any value may be placed. The following statement creates a variable called $testvariable (all variable names in PHP begin with a dollar sign) and assigns it a value of 3:
PHP is a loosely typed language. This means that a single variable may contain any type of data, be it a number, a string of text, or some other kind of value, and may change types over its lifetime. So the following statement, if it appears after the statement above, assigns a new value to our existing $testvariable. In the process, the variable changes type: where it used to contain a number, it now contains a string of text:
$testvariable = "Three";
The equals sign we used in the last two statements is called the assignment operator, as it is used to assign values to variables. Other operators may be used to perform various mathematical operations on values:
$testvariable = 1 + 1; // Assigns a value of 2
$testvariable = 1 - 1; // Assigns a value of 0
$testvariable = 2 * 2; // Assigns a value of 4
$testvariable = 2 / 2; // Assigns a value of 1
Each of the lines above ends with a comment. Comments are a way to describe what your code is doing—they insert explanatory text into your code, and tell the PHP interpreter to ignore it. Comments begin with // and they finish at the end of the same line. You might be familiar with the /* */ style of comment used in other languages—these work in PHP as well. I’ll be using comments
throughout the rest of this book to help explain the code I present.
Now, let’s get back to the four statements above. The operators we used are called the arithmetic operators, and allow you to add, subtract, multiply, and divide numbers. Among others, there is an operator that sticks strings of text together, called the concatenation operator:
$testvariable = "Hi " . "there!";
// Assigns a value of "Hi there!"
Variables may be used almost anywhere that you use an actual value. Consider these examples:
$var1 = 'PHP'; // Assigns a value of 'PHP' to $var1
$var2 = 5; // Assigns a value of 5 to $var2
$var3 = $var2 + 1; // Assigns a value of 6 to $var3
$var2 = $var1; // Assigns a value of 'PHP' to $var2
echo $var1; // Outputs 'PHP'
echo $var2; // Outputs 'PHP'
echo $var3; // Outputs '6'
echo $var1 . ' rules!'; // Outputs 'PHP rules!'
echo "$var1 rules!"; // Outputs 'PHP rules!'
echo '$var1 rules!'; // Outputs '$var1 rules!'
Notice the last two lines in particular. You can include the name of a variable right inside a text string, and have the value inserted in its place if you surround the string with double quotes instead of single quotes. This process of converting variable names to their values is known as variable interpolation. However, as the last line demonstrates, a string surrounded with single quotes will not interpolate
the variable names it contains.
0 comments:
Post a Comment