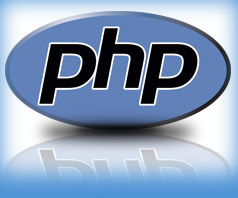
The key to creating interactivity with PHP is to understand the techniques we can use to send information about a user’s interaction along with his or her request for a new Web page. PHP makes this fairly easy, as we’ll now see.
The simplest method we can use to send information along with a page request uses the URL query string. If you’ve ever seen a URL in which a question mark followed the file name, you’ve witnessed this technique in use. Let’s look at an easy example. Create a regular HTML file called welcome1.html (no .php file extension is required, since there will be no PHP code in this file) and insert this
link:
File: welcome.html (excerpt)
<a href="welcome1.php?name=Kevin">Hi, I'm Kevin!</a>
This is a link to a file called welcome1.php, but as well as linking to the file, we’re also passing a variable along with the page request. The variable is passed as part of the query string, which is the portion of the URL that follows the question mark. The variable is called name and its value is Kevin. To restate, we have created a link that loads welcome1.php, and informs the PHP code contained in the file that name equals Kevin.
To really understand the results of this process, we need to look at welcome1.php. Create it as a new HTML file, but, this time, note the .php extension—this tells the Web server that it can expect to interpret some PHP code in the file. In the body of this new file, type the following:
File: welcome1.php (excerpt)
<?php
$name = $_GET['name'];
echo "Welcome to our Website, $name!";
?>
Now, if you use the link in the first file to load this second file, you’ll see that the page says “Welcome to our Website, Kevin!” This is illustrated in Figure 3.1.
Figure 3.1. Greet users with a personalized welcome message.
PHP creates automatically an array variable called $_GET that contains any values passed in the query string. $_GET is an associative array, so the value of the name variable passed in the query string can be accessed as $_GET['name']. Our script assigns this value to an ordinary PHP variable ($name), then displays it as part of a text string using an echo statement.
register_globals before PHP 4.2
In versions of PHP prior to 4.2, the register_globals setting in php.ini was set to On by default. This setting tells PHP to create ordinary variables for all the values supplied in the request automatically. In the previous example, the $name = $_GET['name'];
line would be completely unnecessary if the register_globals setting were set to On, since PHP would do it automatically. Although the convenience of this feature was one aspect that helped to make PHP such a popular language in the first place, novice developers could easily leave security holes in sensitive scripts with it enabled. For a full discussion of the issues surrounding register_globals, see my article
Write Secure Scripts with PHP 4.2![2] at sitepoint.com. You can pass more than one value in the query string. Let’s look at a slightly more complex version of the same example. Change the link in the HTML file to read as follows:
File: welcome2.html (excerpt)
<a href="welcome2.php?firstname=Kevin&lastname=Yank">Hi,
I'm Kevin Yank!</a>
This time, we’ll pass two variables: firstname and lastname. The variables are separated in the query string by an ampersand (&, which is encoded as & as it is a special character in HTML). You can pass even more variables by separating each name=value pair from the next with an ampersand. As before, we can use the two variable values in our welcome2.php file:
File: welcome2.php (excerpt)
<?php
$firstname = $_GET['firstname'];
$lastname = $_GET['lastname'];
echo "Welcome to my Website, $firstname $lastname!";
This is all well and good, but we still have yet to achieve our goal of true user interaction, where the user can enter arbitrary information and have it processed by PHP. To continue with our example of a personalized welcome message, we’d like to allow the user to type his or her name and have it appear in the message.
To allow the user to type in a value, we’ll need to use an HTML form. Here’s the code:
File: welcome3.html (excerpt)
<form action="welcome3.php" method="get">
<label>First Name: <input type="text" name="firstname" />
</label><br />
<label>Last Name: <input type="text" name="lastname" />
</label><br />
<input type="submit" value="GO" />
</form>
This form has the exact same effect as the second link we looked at (with firstname=Kevin&lastname=Yank in the query string), except that you can now enter whatever names you like. When you click the submit button (which is labelled GO), the browser will load welcome3.php and add the variables and their values to the query string for you automatically. It retrieves the names of the variables from the name attributes of the input type="text" tags, and obtains the values from the information the user typed into the text fields. The method attribute of the form tag is used to tell the browser how to send the
variables and their values along with the request. A value of get (as used above) causes them to be passed in the query string (and appear in PHP’s $_GET array), but there is an alternative. It’s not always desirable—or even technically feasible— to have the values appear in the query string. What if we included a textarea tag in the form, to let the user enter a large amount of text? A URL whose
query string contained several paragraphs of text would be ridiculously long, and would possibly exceed the maximum length for a URL in today’s browsers. The alternative is for the browser to pass the information invisibly, behind the scenes. The code for this looks exactly the same, but where we set the form method to get in the last example, here we set it to post:
File: welcome4.html (excerpt)
<form action="welcome4.php" method="post">
<label>First Name:
<input type="text" name="firstname" /></label><br />
<label>Last Name:
<input type="text" name="lastname" /></label><br />
<input type="submit" value="GO" />
</form>
As we’re no longer sending the variables as part of the query string, they no longer appear in PHP’s $_GET array. Instead, they are placed in another array reserved especially for ‘posted’ form variables: $_POST. We must therefore modify welcome4.php to retrieve the values from this new array:
File: welcome4.php (excerpt)
<?php
$firstname = $_POST['firstname'];
$lastname = $_POST['lastname'];
echo "Welcome to my Website, $firstname $lastname!";
?>
The form is functionally identical to the previous one; the only difference is that the URL of the page that’s loaded when the user clicks the GO button will not have a query string. On the one hand, this lets you include large values, or sensitive values (like passwords), in the data that’s submitted by the form, without their appearing in the query string. On the other hand, if the user bookmarks the page that results from the form’s submission, that bookmark will be useless, as it doesn’t contain the submitted values. This, incidentally, is the main reason that search engines use the query string to submit search terms. If you bookmark a search results page on Google[3] or AltaVista[4], you can use that bookmark to perform the same search again later, because the search terms are contained in the URL. Sometimes, you want access to a variable without having to worry about whether it was sent as part of the query string or a form post. In cases like these, the special $_REQUEST array comes in handy. It contains all the variables that appear in both $_GET and $_POST. With this variable, we can modify our form processing script one more time so that it can receive the first and last names of the user from either source:
File: welcome5.php (excerpt)
<?php
$firstname = $_REQUEST['firstname'];
$lastname = $_REQUEST['lastname'];
echo "Welcome to my Website, $firstname $lastname!";
?>
That covers the basics of using forms to produce rudimentary user interaction with PHP. I’ll cover more advanced issues and techniques in later examples.
0 comments:
Post a Comment